Unity 入门
- MonoBehavior 基类
- 生命周期函数
- 获取脚本
- GameObject
-
- 时间相关 API
-
- Vector3
- Position
- 位移
- EulerAngles
- 缩放
-
- 父子关系
- 坐标转换
- 世界坐标系 转 本地坐标系
- 本地坐标系 转 世界坐标系
- Input
-
- Camera
-
- 碰撞检测
- Rigidbody 刚体
- Collider
- 物理材质
- 常用函数
-
- 刚体加力
- 方法
- 添加水平力
- 添加扭矩力
- 改变速度
- 给世界坐标系的点加力
- 力的模式
- 自带的力相关脚本
- 刚体休眠
MonoBehavior 基类
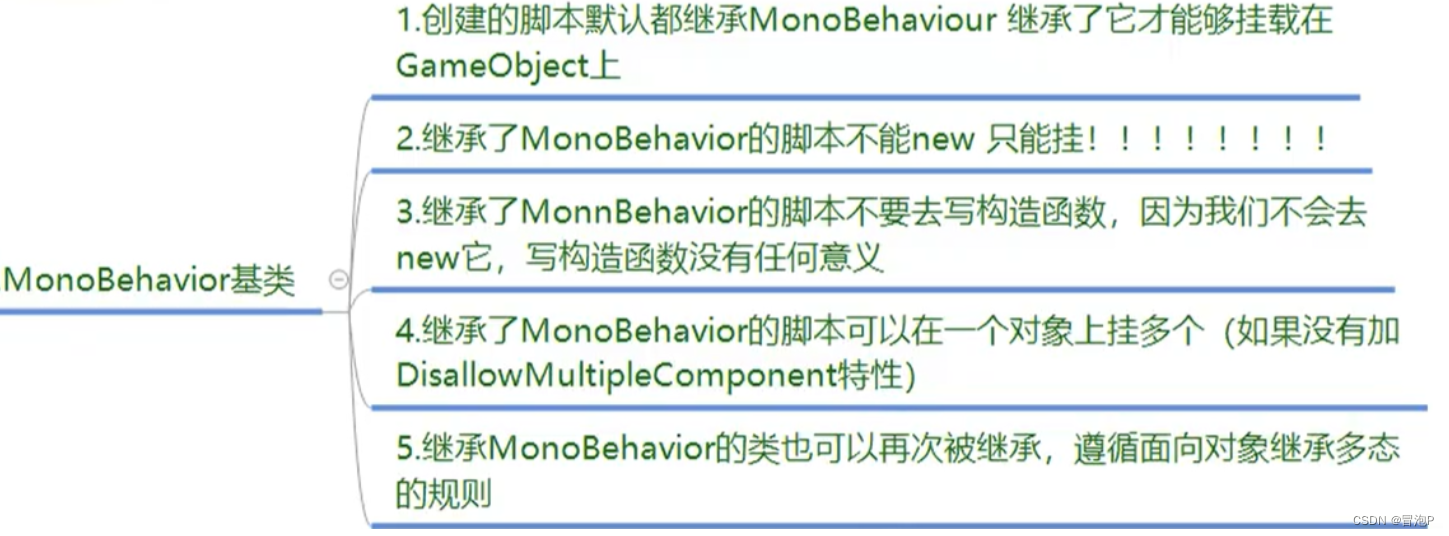
生命周期函数
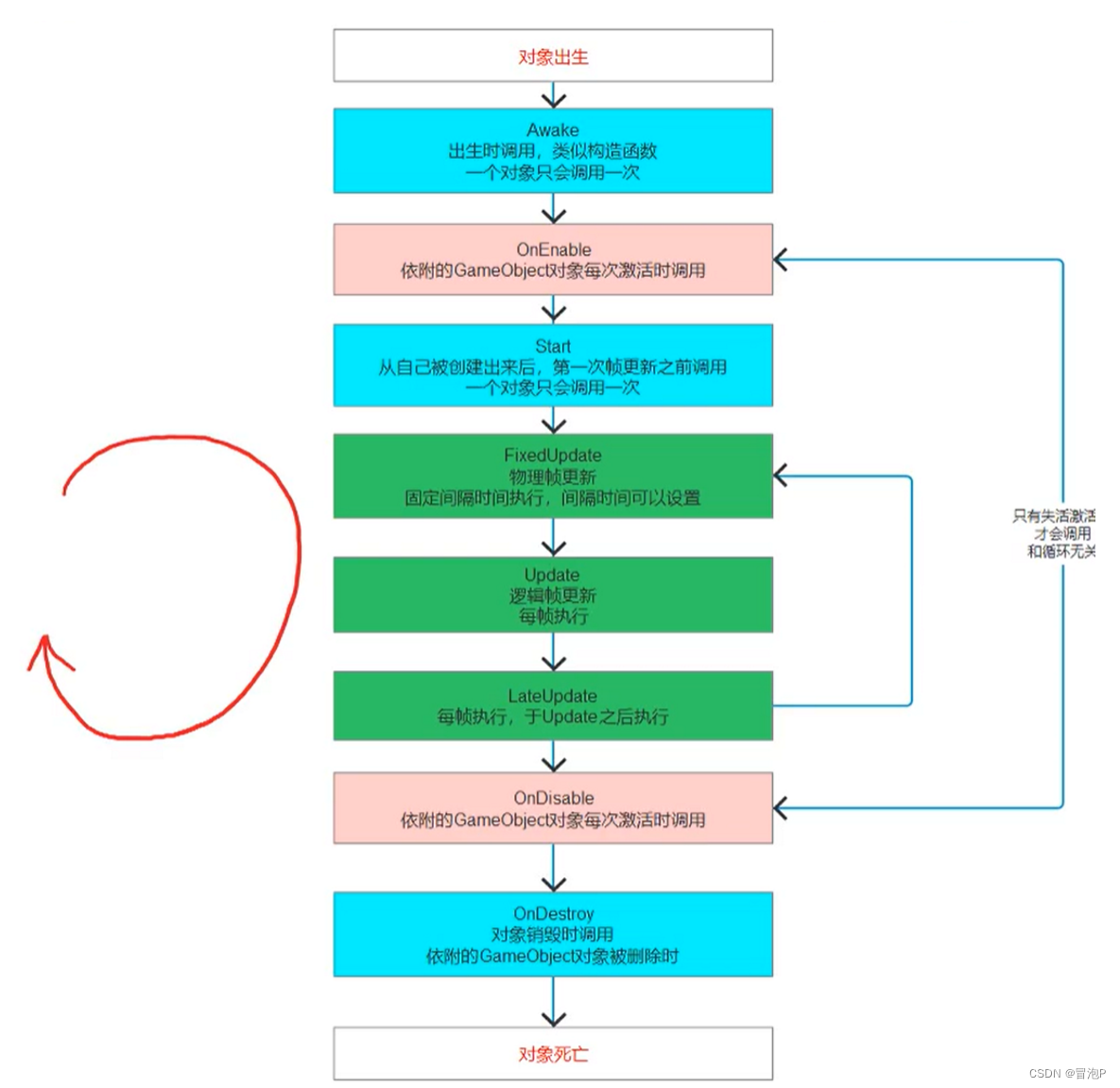
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| public class test : MonoBehaviour { private void Awake() { print("Awake!!"); }
private void OnEnable() { print("OnEnable!!"); }
void Start() { print("Start!!"); }
private void FixedUpdate() { print("FixedUpdate"); }
private void Update() { print("Update"); }
private void LateUpdate() { print("LateUpdate"); }
private void OnDisable() { print("OnDisable"); }
private void OnDestroy() { print("OnDestroy!!"); } }
|
获取脚本
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| otherTest myot = this.GetComponent<otherTest>(); print("my Component :" + myot);
otherTest ot = obj.GetComponent<otherTest>(); print("other Component :" + ot);
otherTest childrenOT = this.GetComponentInChildren<otherTest>(); print("children Component :" + childrenOT);
otherTest ParentOT = this.GetComponentInParent<otherTest>(); print("parent Component :" + ParentOT);
otherTest tryOT; if (this.TryGetComponent<otherTest>(out tryOT)) { print("获取成功"); }
|
GameObject
一些属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| this.enabled = true;
bool isActive = this.gameObject.activeSelf;
bool isStatic = this.gameObject.isStatic;
string tag = this.gameObject.tag;
int layer = this.gameObject.layer;
|
静态方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| GameObject obj = GameObject.CreatePrimitive(PrimitiveType.Cube);
GameObject.Find("otherTest"); GameObject.FindGameObjectWithTag("Player"); GameObject[] objs = GameObject.FindGameObjectsWithTag("Player");
GameObject instObj = GameObject.Instantiate(testOBJ);
GameObject.Destroy(instObj); GameObject.Destroy(instObj,5); GameObject.Destroy(this); GameObject.DontDestroyOnLoad(hsgmOjc) /过景移对,里不除载本象
|
成员方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| GameObject gameObject = new GameObject(); GameObject gameObject2 = new GameObject("Test"); GameObject gameObject3 = new GameObject("TestAddComponent",typeof(otherTest));
otherTest ot = this.AddComponent<otherTest>();
bool isTag = this.CompareTag("Player");
bool isActive = this.gameObject.activeSelf; this.gameObject.SetActive(true);
|
时间相关 API
时间缩放
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| void Start() { Time.timeScale = 0; Time.timeScale = 2;
float deltaTime = Time.deltaTime; float unscaleDelatTime = Time.unscaledDeltaTime;
float time = Time.time; float unscaledTime = Time.unscaledTime;
float frameCount = Time.frameCount;
}
private void FixedUpdate() { float fixedDeltaTime = Time.fixedDeltaTime; float fixedUnscaledDelateTime = Time.fixedUnscaledDeltaTime; }
|
Unity
中的坐标系与 UE
不同,垂直屏幕向里面是 z轴
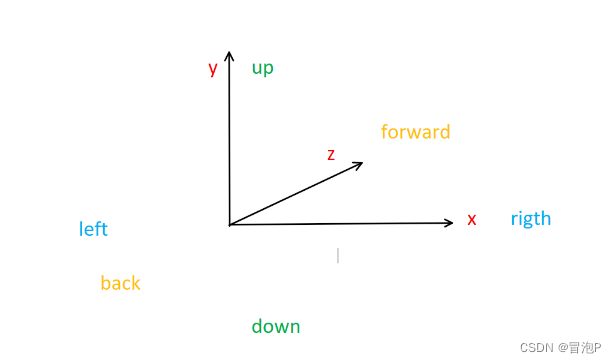
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| Vector3 v1 = new Vector3(1, 2); Vector3 v2 = new Vector3(1, 5, 3);
Vector3 zero = Vector3.zero; Vector3 right = Vector3.right; Vector3 left = Vector3.left; Vector3 forward = Vector3.forward; Vector3 back = Vector3.back; Vector3 up = Vector3.up; Vector3 down = Vector3.down;
float distance = Vector3.Distance(v1, v2);
|
Position
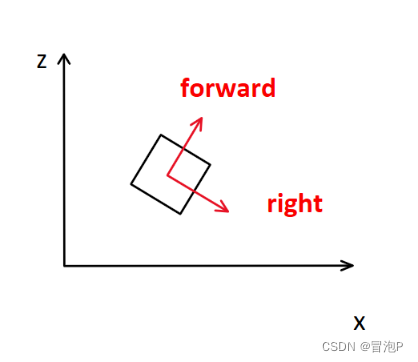
1 2 3 4 5 6 7
| Vector3 postiontion = this.transform.position; Vector3 localPosition = this.transform.localPosition; this.transform.position = new Vector3(1, 2, 3);
Vector3 myForward = this.transform.forward;
|
位移
1 2 3 4 5 6 7 8
| void Update() { this.transform.position += this.transform.forward * Time.deltaTime; this.transform.Translate(Vector3.forward * Time.deltaTime); this.transform.Translate(this.transform.forward * Time.deltaTime, Space.World); }
|
EulerAngles
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| void Update() { Vector3 eulerAngles = this.transform.eulerAngles; Vector3 localEulerAngles = this.transform.localEulerAngles;
this.transform.Rotate(Vector3.up * Time.deltaTime * 10); this.transform.Rotate(Vector3.up * Time.deltaTime * 10, Space.World);
this.transform.RotateAround(Vector3.zero, Vector3.up, 10 * Time.deltaTime); }
|
缩放
一般改localScale
,相对于父对象的缩放,即相对缩放
1 2 3
| Vector3 lossyScale = this.transform.lossyScale; Vector3 localScale = this.transform.localScale;
|
看向
1 2 3 4 5 6 7 8
| public Transform lookObject; void Start() { this.transform.LookAt(Vector3.zero); this.transform.LookAt(lookObject); }
|
父子关系
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public Transform son; void Start() { print(this.transform.parent); this.transform.parent = null; this.transform.parent = GameObject.Find("Cube").transform; this.transform.SetParent(null); this.transform.SetParent(GameObject.Find("Cube").transform, true); this.transform.SetParent(GameObject.Find("Cube").transform, true);
this.transform.DetachChildren(); this.transform.Find("Cube");
for(int i = 0; i < this.transform.childCount; i++) { print(this.transform.GetChild(i)); }
son.IsChildOf(this.transform); son.GetSiblingIndex(); son.SetAsFirstSibling(); son.SetAsLastSibling(); son.SetSiblingIndex(2);
}
|
坐标转换
世界坐标系 转 本地坐标系
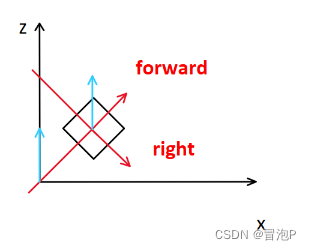
1 2 3 4 5
| print("(0,0,1)点相对于本地坐标系的位置:" + this.transform.InverseTransformPoint(Vector3.forward));
print("(0,0,1)方向相对于本地坐标系的方向:" + this.transform.InverseTransformVector(Vector3.forward));
|
本地坐标系 转 世界坐标系
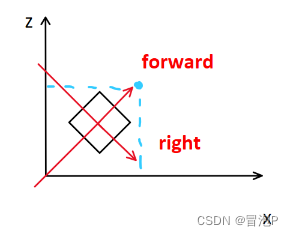
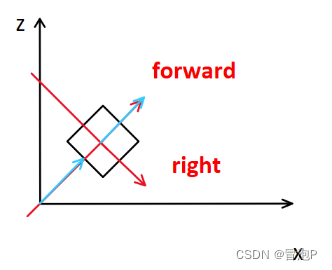
1 2 3 4 5
| print(this.transform.TransformPoint(Vector3.forward));
print(this.transform.TransformDirection(Vector3.forward)); print(this.transform.TransformVector(Vector3.forward));
|
鼠标相关
1 2 3 4 5 6 7 8 9 10 11 12 13
| Vector3 mousePosition = Input.mousePosition;
bool isButtonDown = Input.GetMouseButtonDown(0);
bool isButtonUp = Input.GetMouseButtonUp(0);
bool isButton = Input.GetMouseButton(0);
Vector2 mouseScrollDelta = Input.mouseScrollDelta;
|
键盘相关
1 2 3 4
| bool isKeyDown = Input.GetKeyDown(KeyCode.W); bool isKey = Input.GetKey(KeyCode.W); bool isKeyUp = Input.GetKeyUp(KeyCode.W);
|
默认轴输入
1 2 3 4 5
| float axisHorizontal = Input.GetAxis("Horizontal"); float axisVertical = Input.GetAxis("Vertical"); float mouseX = Input.GetAxis("Mouse X"); float mouseY = Input.GetAxis("Mouse Y");
|
另外一种:GetAxisRaw
方法取值有 -1 0 1 没有浮点过度
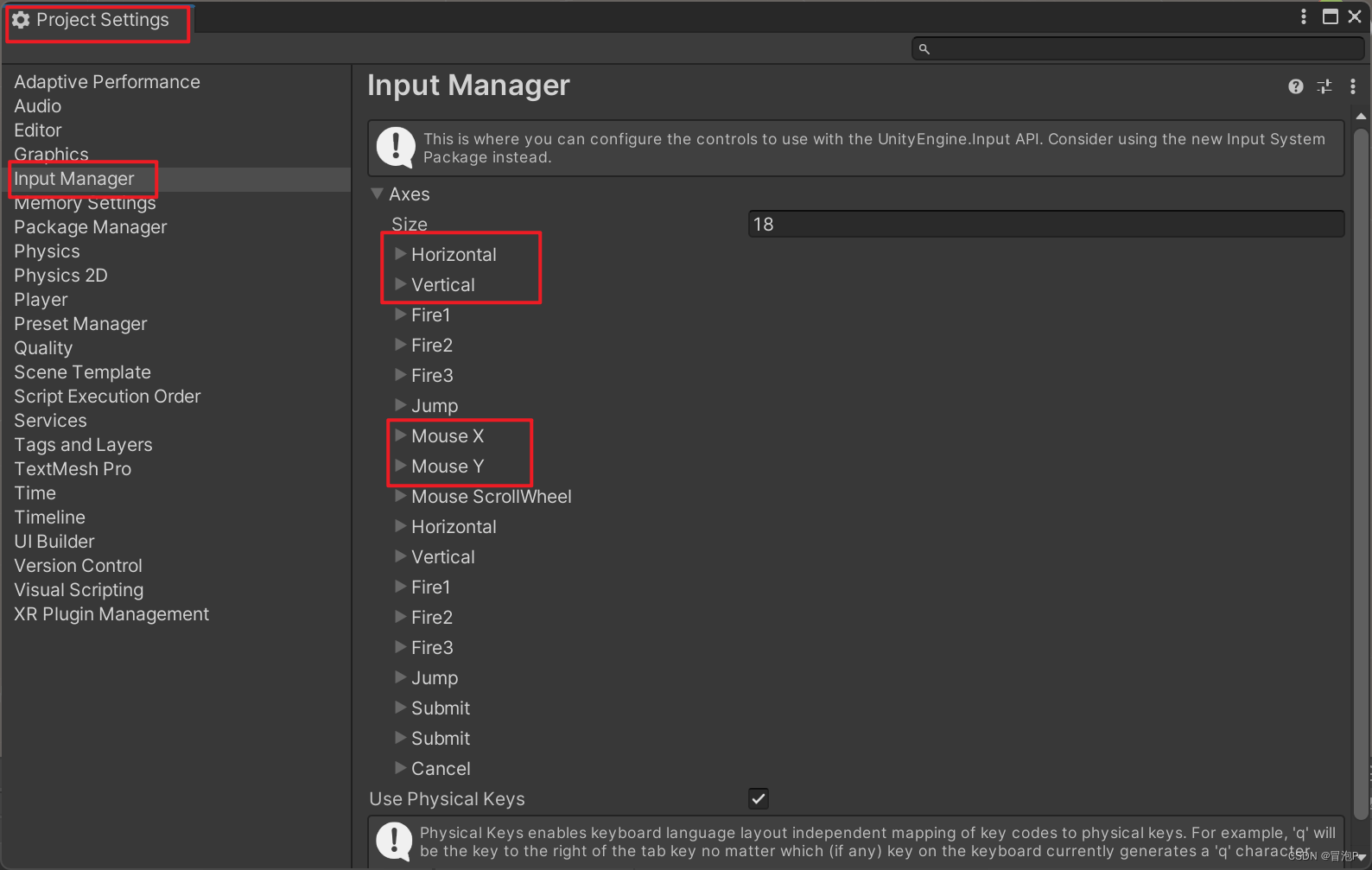
其他
1 2 3 4 5 6 7 8 9
| bool anKeyDown = Input.anyKeyDown;
bool anyKey = Input.anyKey;
if (Input.anyKeyDown) { string inputString = Input.inputString; }
|
Camera
参数
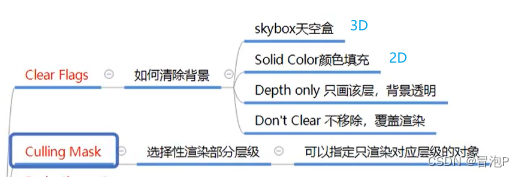
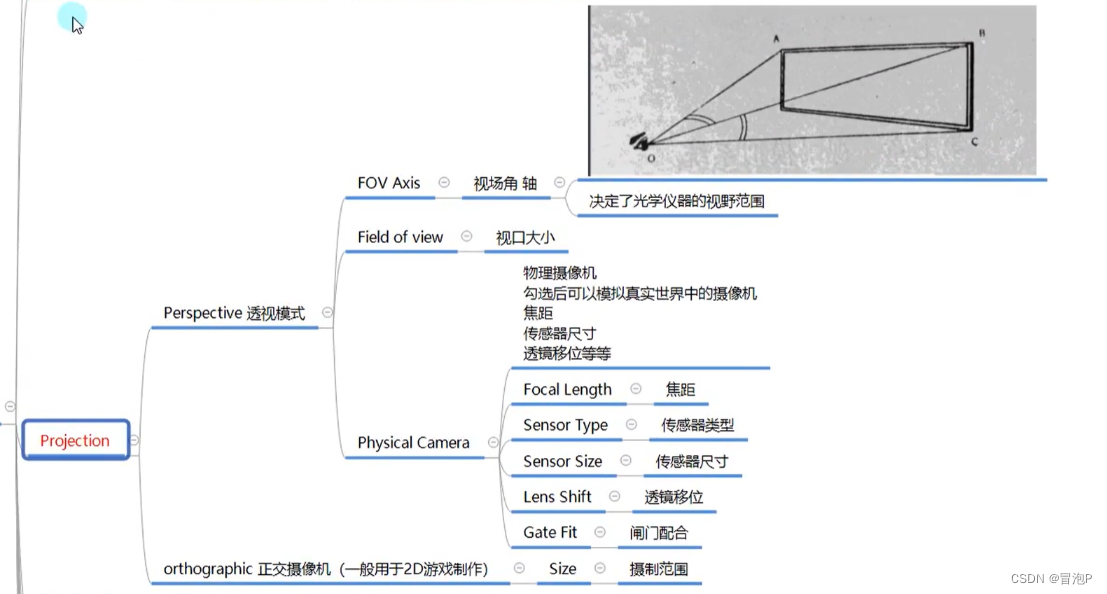
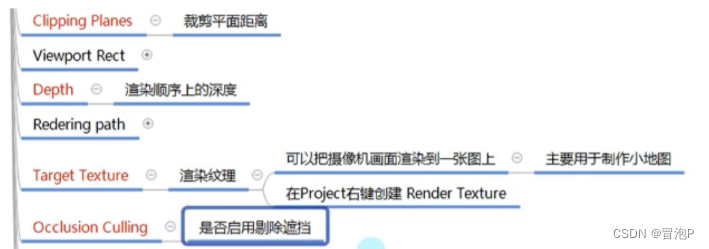
成员
1 2 3 4 5 6 7 8 9 10 11
|
Camera camera = Camera.main;
Camera[] allCameras = Camera.allCameras;
Vector3 screenPoint = Camera.main.WorldToScreenPoint(this.transform.position);
Vector3 worldPoint = Camera.main.ScreenToWorldPoint(Input.mousePosition);
|
世界坐标 转 屏幕坐标 Vector3:x , y 表示屏幕坐标系,z 表示相对于摄像机的纵深距离
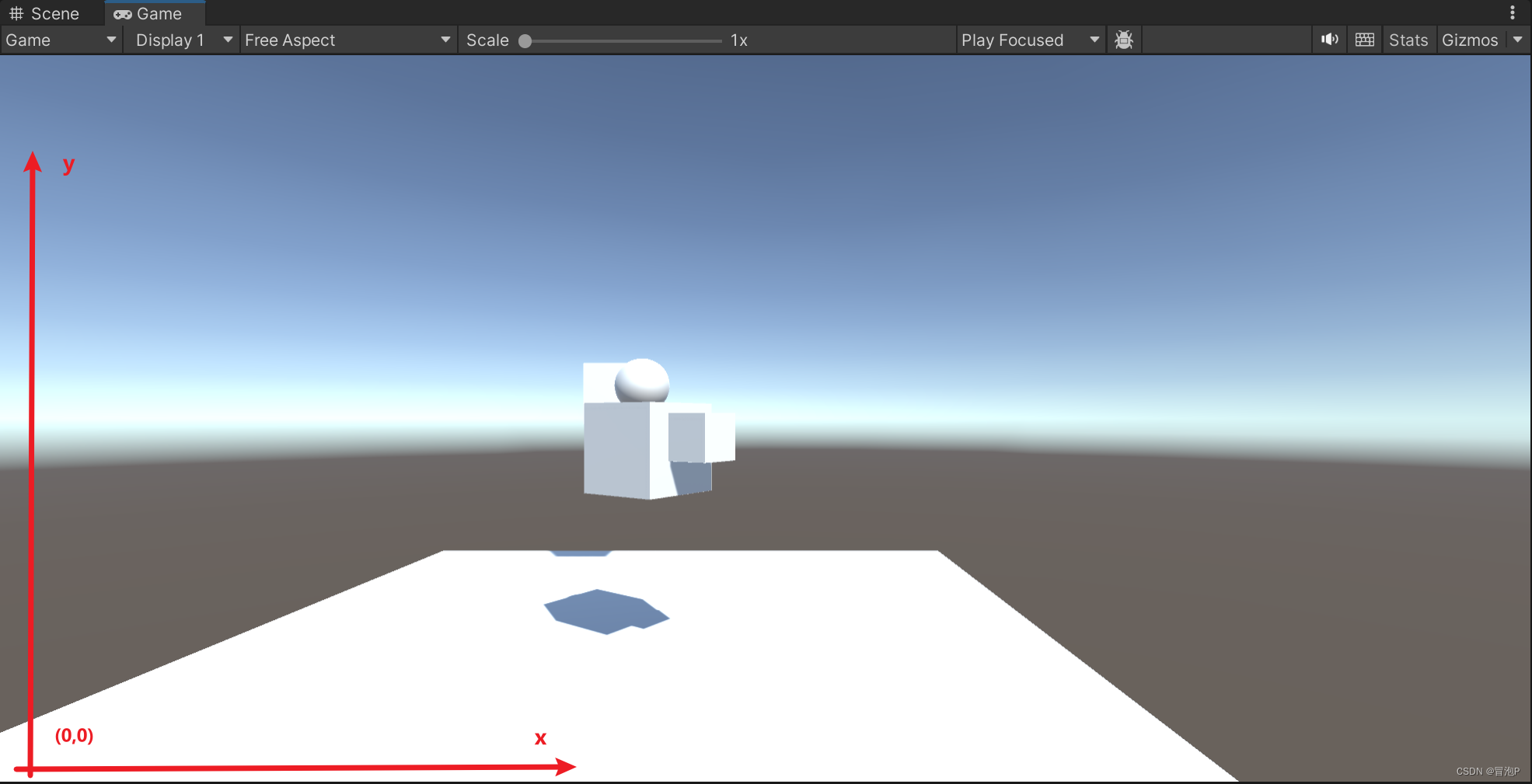
屏幕坐标 转 世界坐标 Vector3: z 表示纵深距离,类似横切面
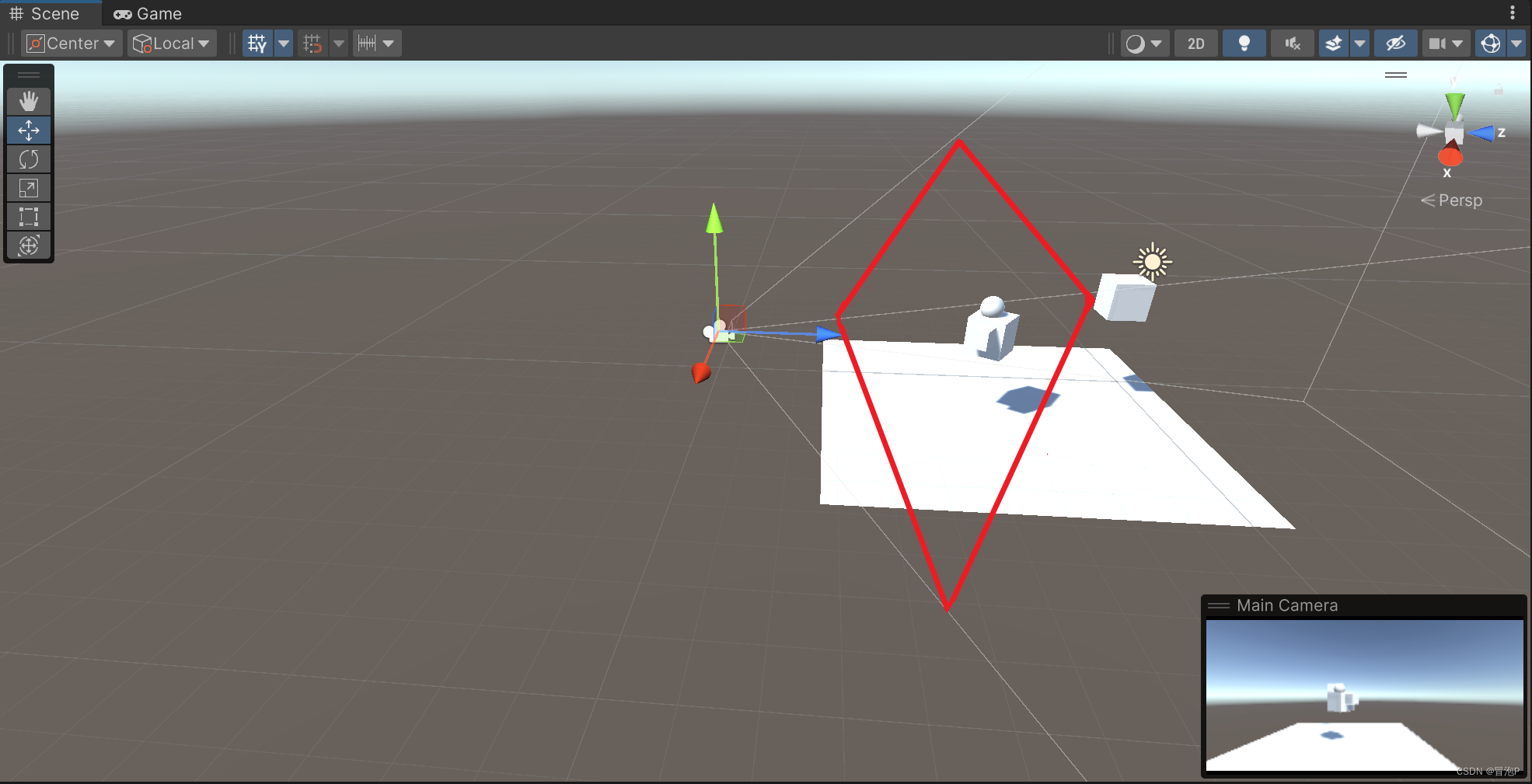
碰撞检测
Rigidbody 刚体
两个物体相互碰撞必要条件:1.都有碰撞盒子 2.至少一个拥有刚体
刚体类似于模拟物理
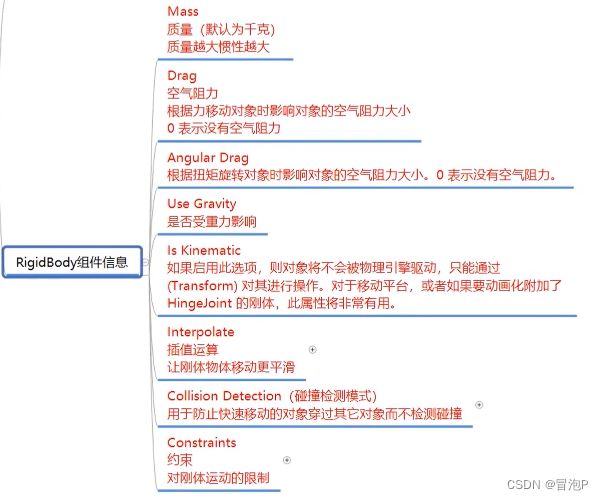
详细:
物理帧更新直接影响刚体插值运算
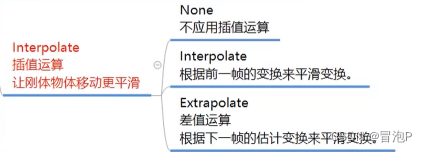
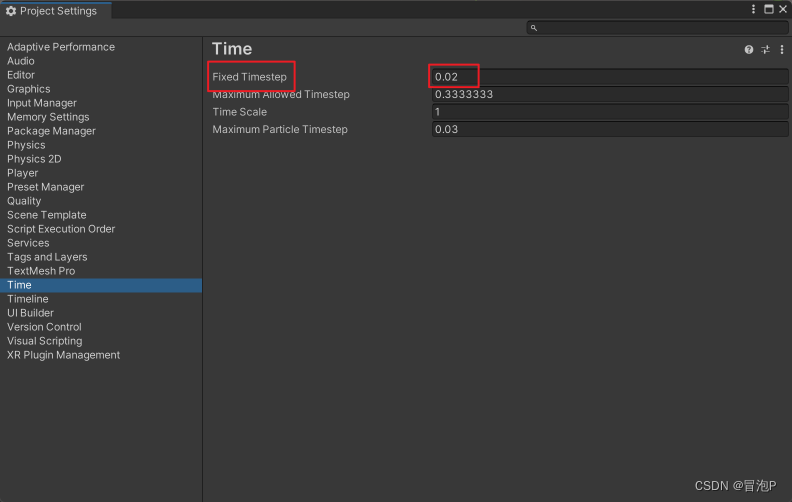
碰撞检测模式,具体含义不重要,了解性能消耗大小即可
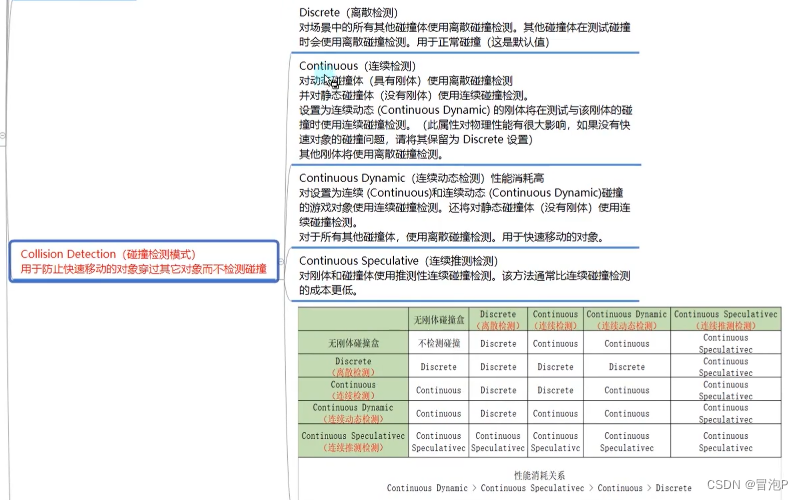
约束轴移动或者轴旋转
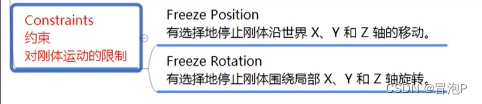
Collider
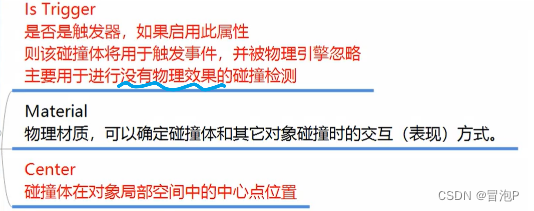
物理材质
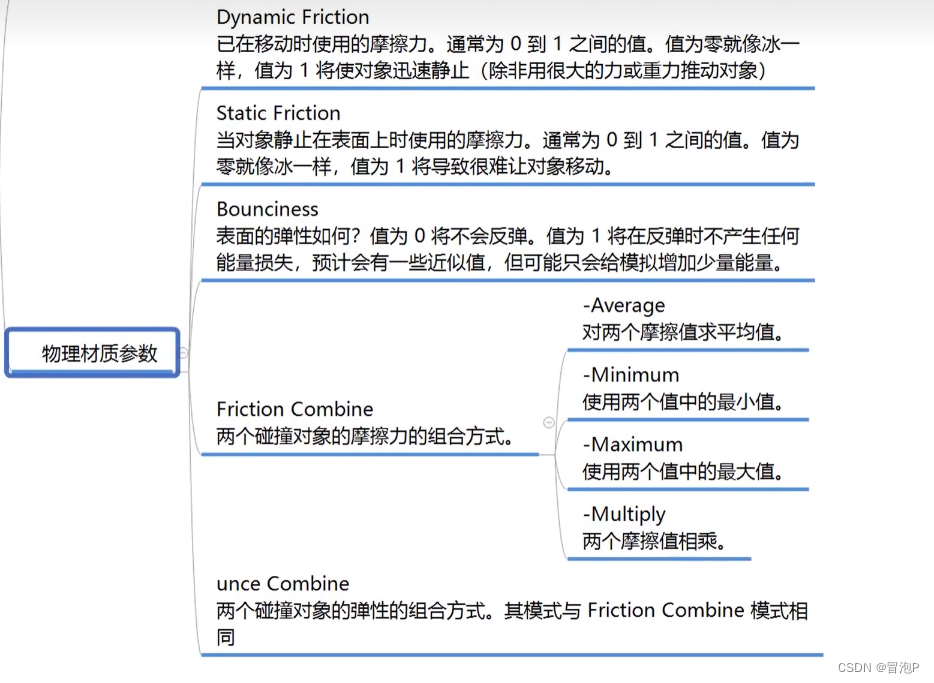
常用函数
特殊的生命周期
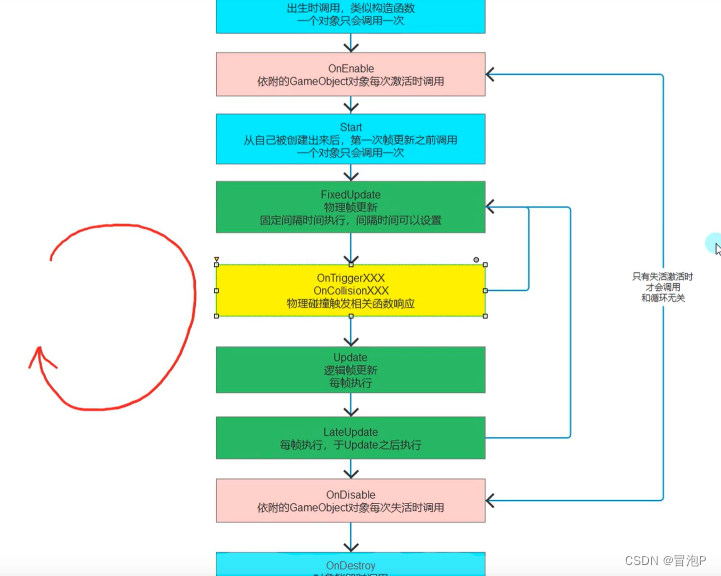
物理碰撞检测
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| private void OnCollisionEnter(Collision other) { GameObject gameObject = other.gameObject; Collider collider = other.collider; Transform transform = other.transform; }
private void OnCollisionStay(Collision other) {
}
private void OnCollisionExit(Collision other) {
}
|
触发器检测
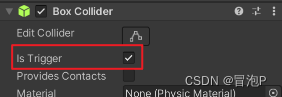
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| private void OnTriggerEnter(Collider other) {
}
private void OnTriggerStay(Collider other) {
}
private void OnTriggerExit(Collider other) {
}
|
其他注意

刚体加力
方法
获取刚体组件
1
| Rigidbody rigidBody = this.gameObject.GetComponent<Rigidbody>();
|
添加水平力
1 2 3 4
| rigidBody.AddForce(Vector3.forward);
rigidBody.AddRelativeForce(Vector3.forward);
|
添加扭矩力
1 2 3 4
| rigidBody.AddTorque(Vector3.up);
rigidBody.AddRelativeTorque(Vector3.up);
|
改变速度
1
| rigidBody.velocity = Vector3.forward;
|
给世界坐标系的点加力
1 2 3 4
| float forceValue = 100; float radius = 10; Vector3 point = Vector3.zero; rigidBody.AddExplosionForce(forceValue,point,radius);
|
力的模式

自带的力相关脚本
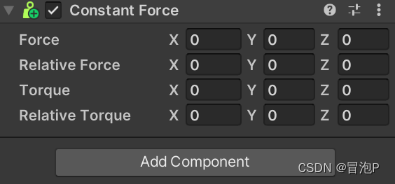
刚体休眠
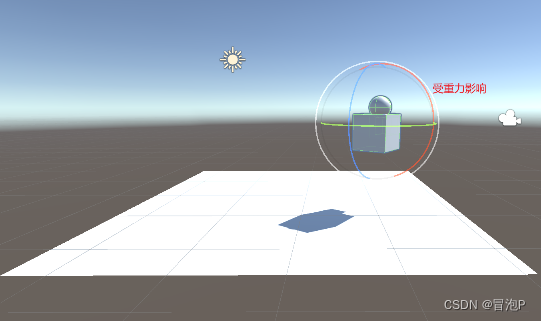
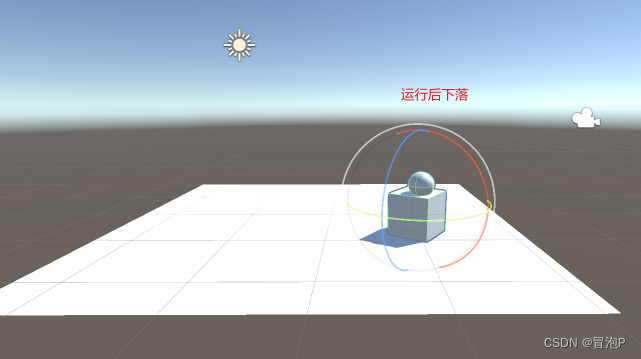
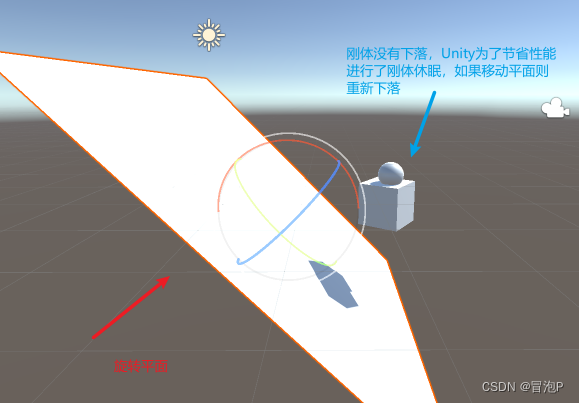
1 2 3 4 5 6
| if (rigidBody.IsSleeping()) { rigidBody.WakeUp(); } ```
|